Okay, here’s my blog post about “ajax vs”, written from my personal experience:

So, I was messing around with this web project, you know, trying to make things feel all smooth and interactive. The big question was, how do I get data from the server without reloading the whole darn page? That’s where the “ajax” thing comes in… or does it?
I remember banging my head against the wall for hours. I started by Googling, “how to update part of a page javascript,” or something like that. I kept seeing “AJAX” pop up, and honestly, it sounded intimidating.
Digging into AJAX (the old way)
First, I tried the “classic” AJAX way. You know, the one with the XMLHttpRequest object. It looked something like this:
- Create a new XMLHttpRequest.
- Set up an onreadystatechange function. This is where things get…funky. You’re basically saying, “Hey, when the server responds, do this stuff.”
- Check the readyState and status. If readyState is 4 and status is 200, that means the response is good to go.
- Inside that if statement, you finally get to do something with the data you got back (usually with responseText or responseXML).
- Then you open() the request, specifying the method (“GET” or “POST”) and the URL.
- Finally, you send() the request.
I copied and pasted some code, tweaked it a bit, and…it kinda worked! I managed to update a little <div> with some text from the server. It felt like magic, but also, a bit clunky. All those steps! All those properties to check!
Is the fetch() more easy?
Then, someone in a forum mentioned “fetch().” Apparently, it’s a newer, “easier” way to do the same thing. I was skeptical, but hey, I was already down the rabbit hole.
So, I tried this “fetch()” thing. The code looked way simpler:
- Call fetch() with the URL.
- Use .then() to handle the response. The response comes in as a “Response” object.
- Call .json() (if you’re expecting JSON) or .text() (if you’re expecting plain text) on the Response object. This returns another promise!
- Use another .then() to actually do something with the parsed data.
- (Optional) Use .catch() to handle any errors.
I did it! Using fetch(), and it felt more intuitive. The code was cleaner, and it handled errors in a more straightforward way. It’s like they took all the messy parts of XMLHttpRequest and wrapped them up in a nice, neat package.
So, Which is winner?
For my simple project, fetch() was the clear winner. It was easier to read, easier to write, and just felt…better. But, I can see why the old XMLHttpRequest way is still around. Maybe it’s needed for some super specific situations, or maybe some older websites just haven’t been updated yet.
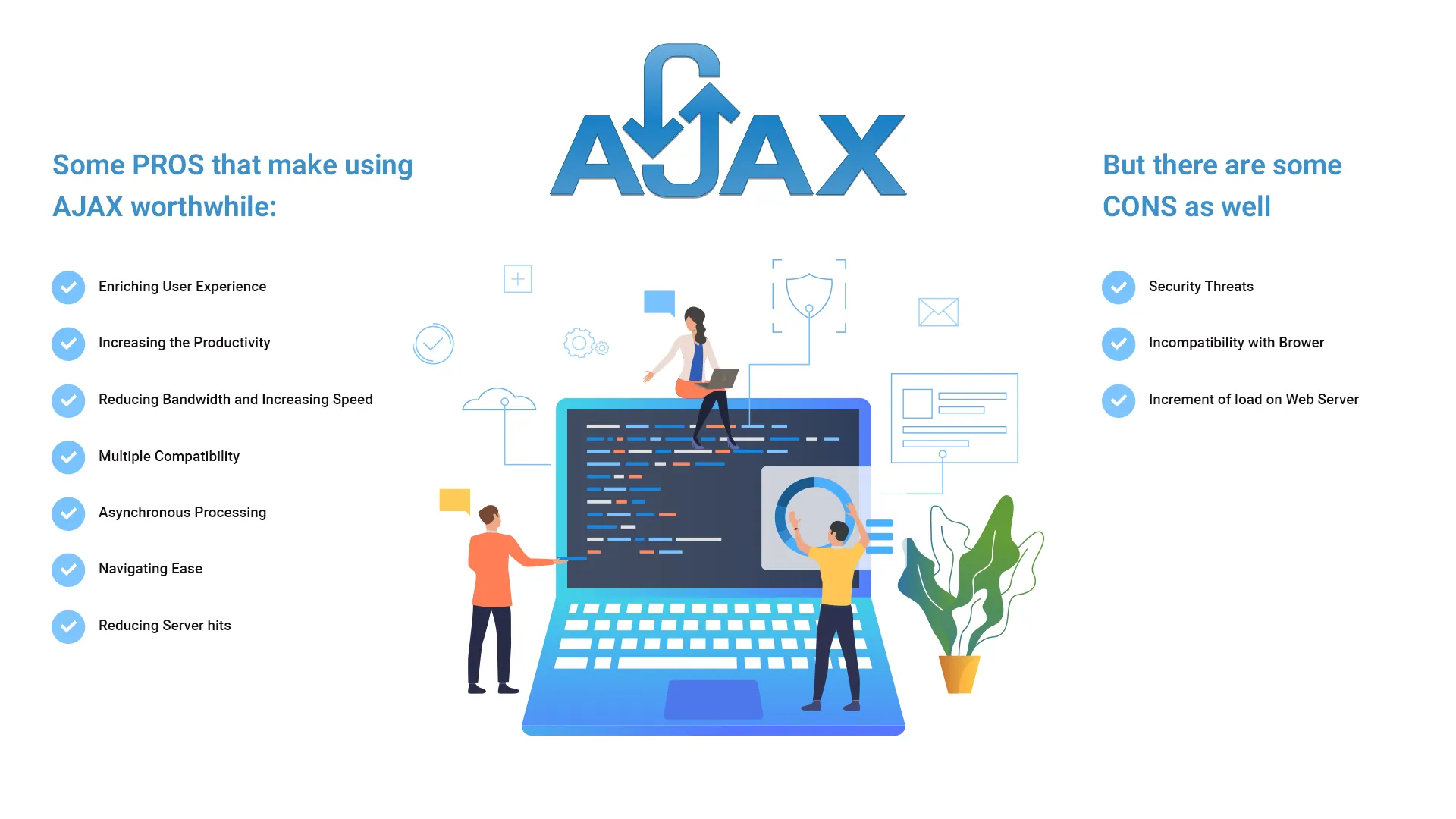
I’m going to keep practicing with “fetch()” for the data transfer, and see how it goes from there!