Okay, so I wanted to mess around with variable arguments in C, you know, those functions that can take a bunch of inputs without you having to specify how many beforehand. I’ve seen them around, like in `printf`, and I was curious how they actually work under the hood.
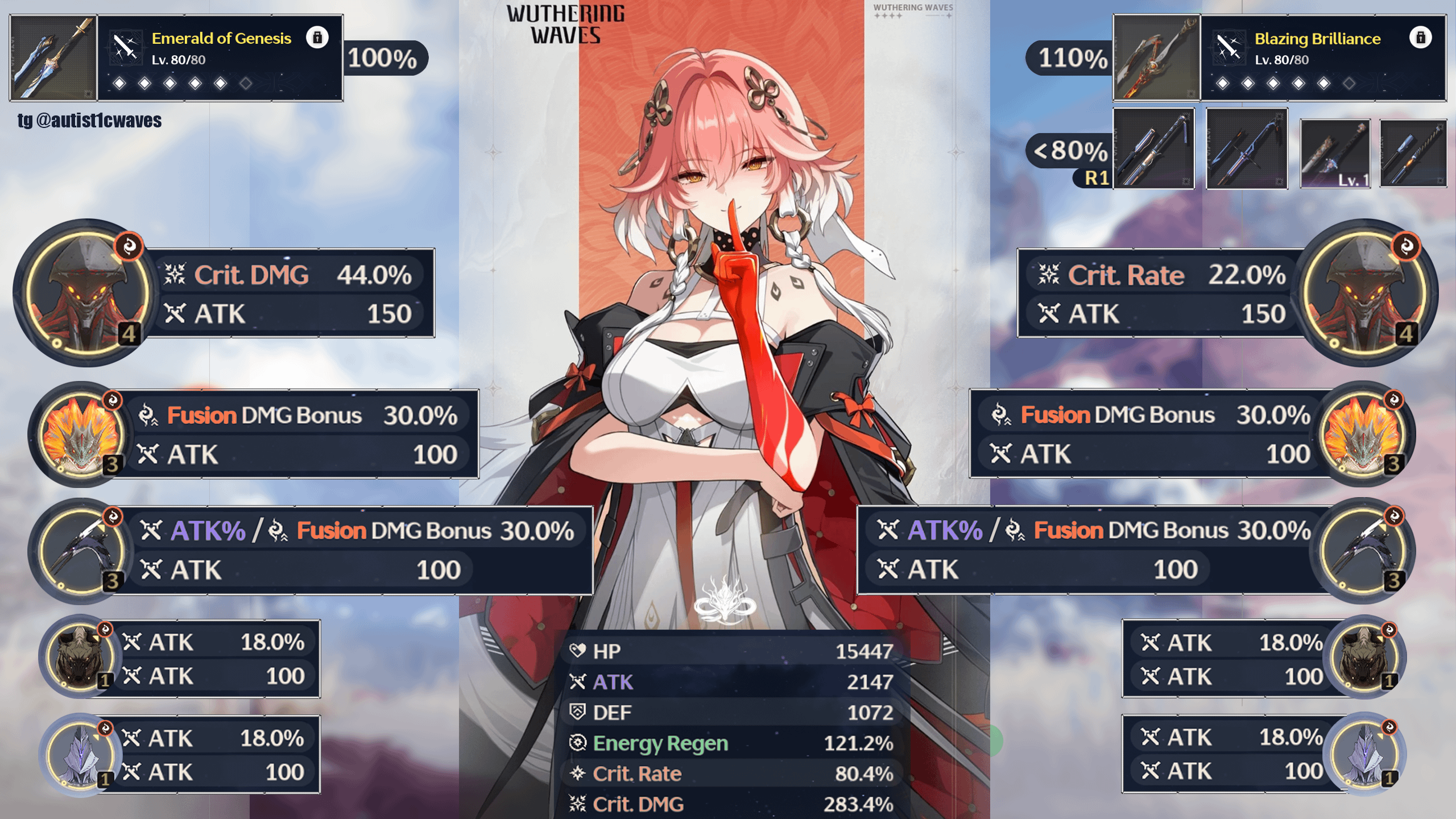
Getting Started
First thing I did was fire up my trusty code editor and include the “ header file. This bad boy is essential because it gives you all the tools you need to play with variable arguments.
Setting Up the Function
I decided to make a simple function called `sum_up` that would just add up all the numbers I throw at it. Here’s how I set it up:
int sum_up(int count, ...) {
// Your code goes here
See that `…`? That’s the magic that tells the compiler, “Hey, this function can take more arguments than I’ve explicitly listed here.” The `count` parameter is there to tell the function how many numbers we’re actually passing in.
Diving into the `va_list`
Inside the function, the first thing you need is a `va_list` variable. Think of it as a pointer that will help you walk through your arguments. You declare it like this:
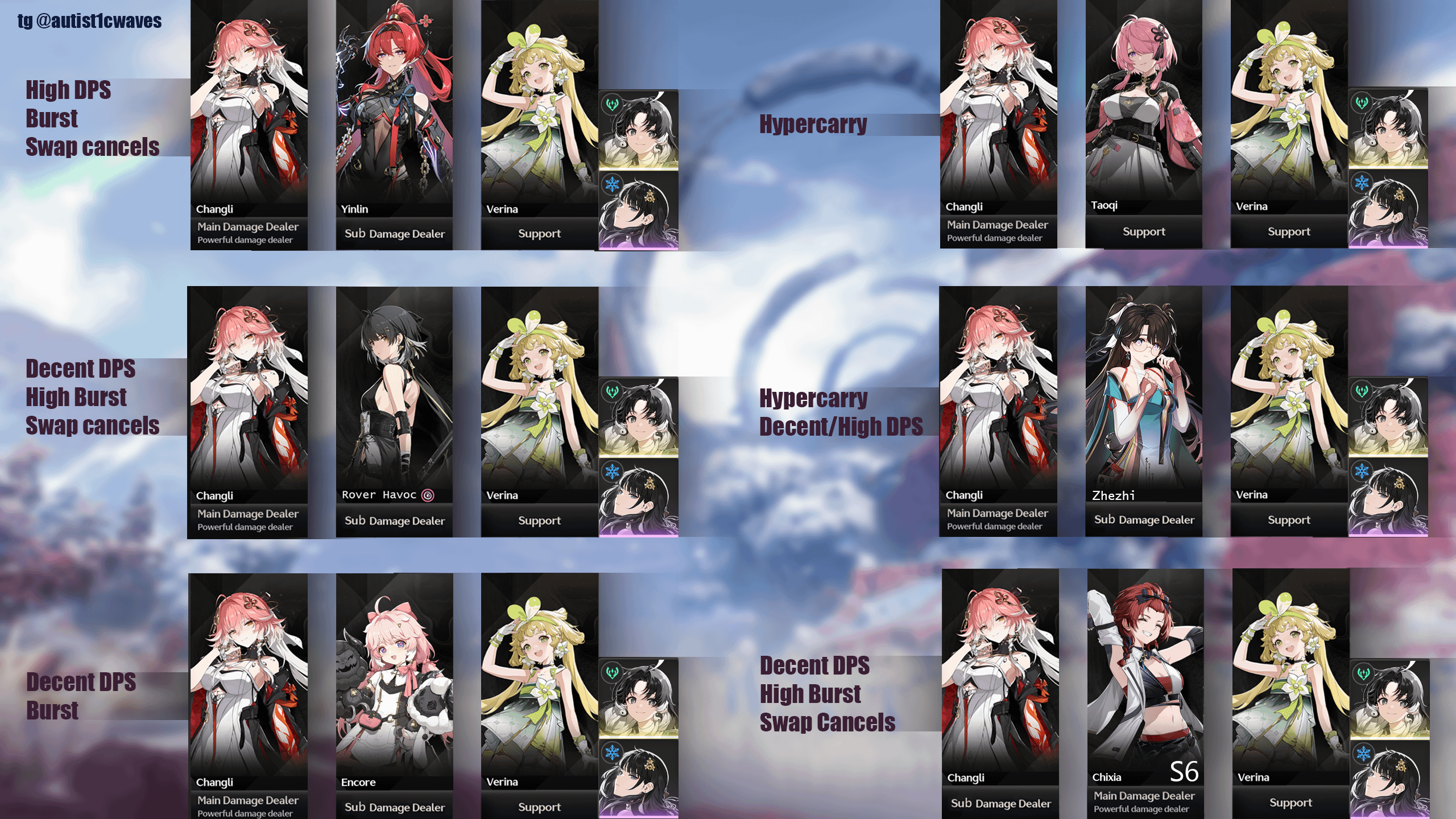
va_list my_numbers;
Initializing and Accessing the Arguments
Before you can use your `va_list`, you gotta initialize it with `va_start`. This macro takes two things: your `va_list` variable and the name of the last named parameter before the `…`. In our case, it’s `count`.
va_start(my_numbers, count);
Now, to grab each argument, you use `va_arg`. This macro also needs two things: your `va_list` and the type of the argument you expect. Since I’m summing integers, I used `int`.
The Loop
I set up a `for` loop to go through the numbers. Inside the loop, I used `va_arg(my_numbers, int)` to get each number and added it to a running total.
int total = 0;
for (int i = 0; i < count; i++) {
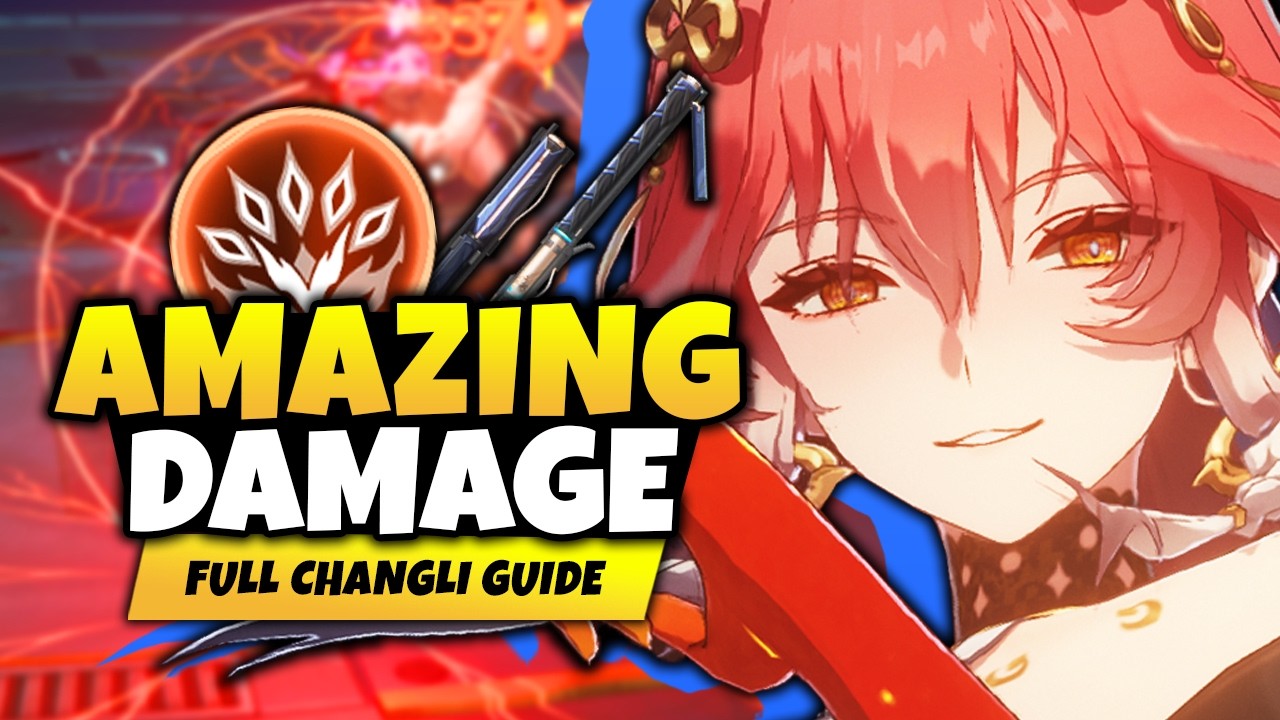
total += va_arg(my_numbers, int);
Cleaning Up
After you’re done with your arguments, you have to call `va_end`. It’s like closing a file – it cleans up the `va_list` and keeps things tidy.
va_end(my_numbers);
The Result
Finally, I returned the `total` from my function. And that’s it! I wrote a simple function that can sum up any number of integers.
int sum_up(int count, ...) {
va_list my_numbers;
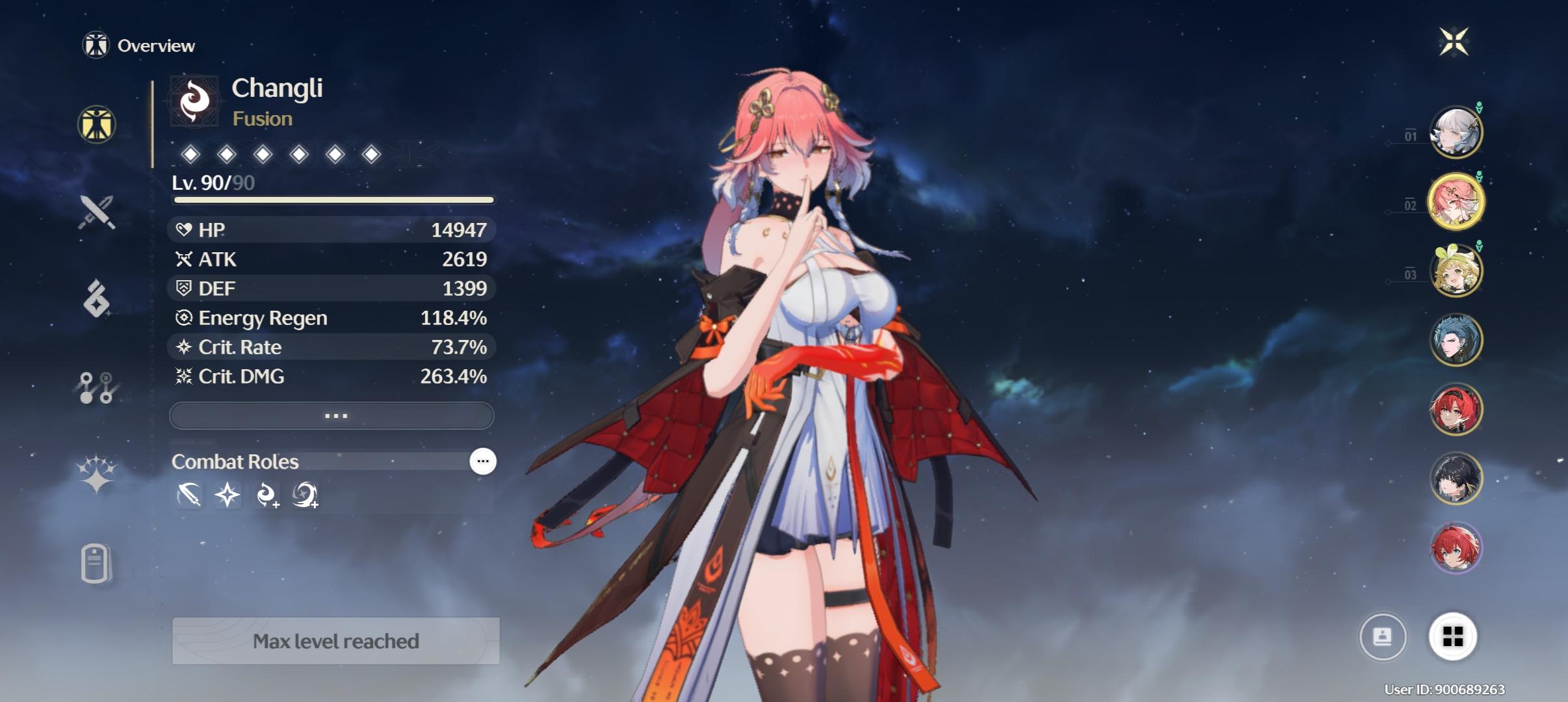
va_start(my_numbers, count);
int total = 0;
for (int i = 0; i < count; i++) {
total += va_arg(my_numbers, int);
}
va_end(my_numbers);
return total;
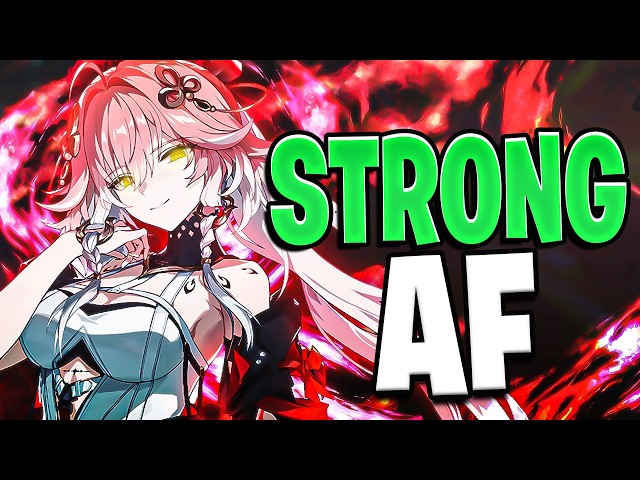
Trying It Out
I tested my function by calling it with different numbers of arguments.
int main() {
printf("Sum is %dn", sum_up(3, 1, 2, 3)); // Should print 6
printf("Sum is %dn", sum_up(5, 10, 20, 30, 40, 50)); // Should print 150
return 0;
I compiled the code and ran it, and guess what? It worked perfectly! I saw the correct sums printed on the screen. It’s pretty satisfying to see your code working as intended, you know?
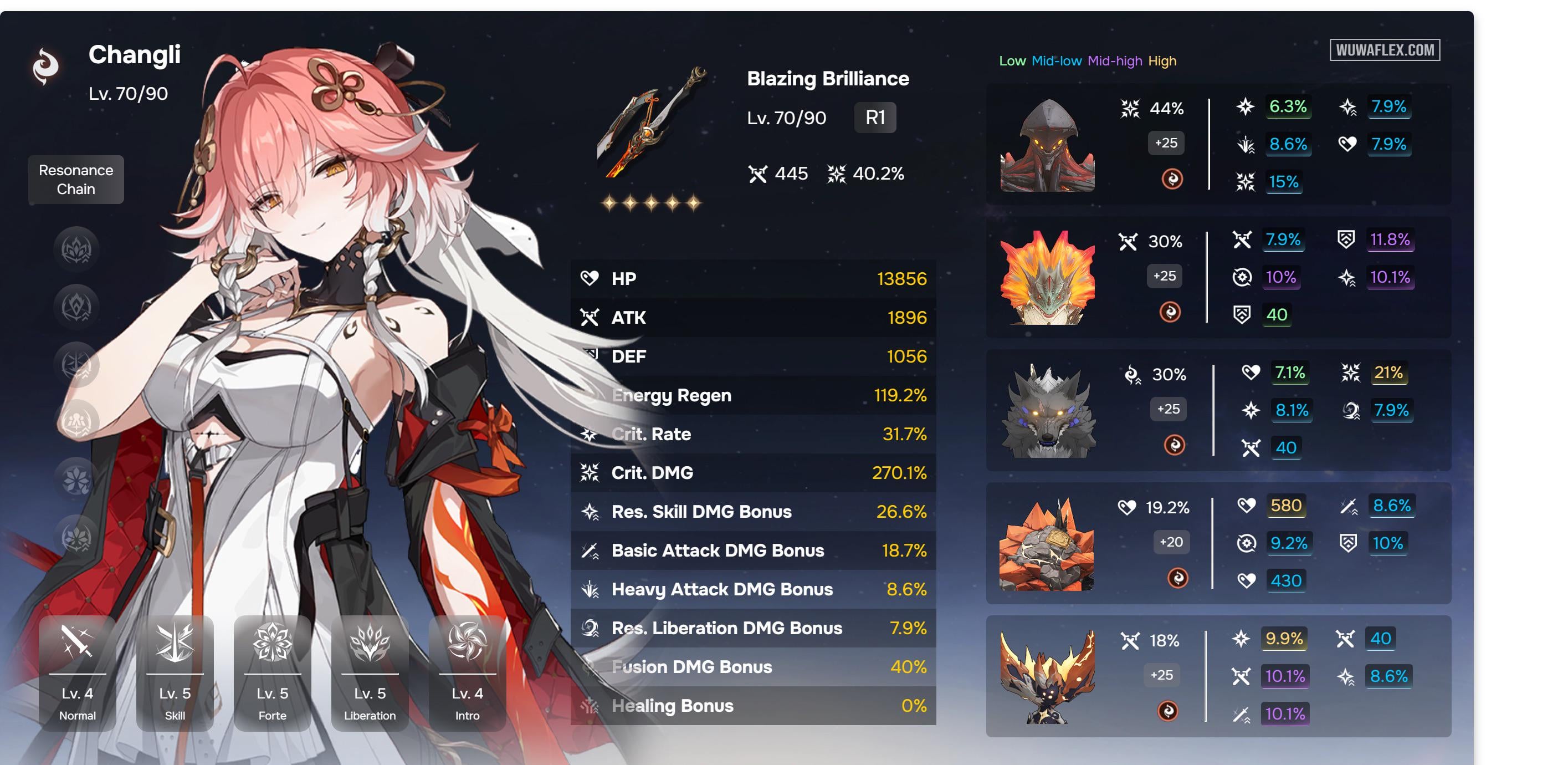
So, there you have it. That’s how I learned about variable arguments in C and wrote a function to sum up numbers. It’s a neat trick to have up your sleeve, and it’s simpler than it looks once you get the hang of it.