Okay, so yesterday I was messing around trying to get some data on the Philadelphia Phillies vs. Washington Nationals game. It wasn’t as straightforward as I thought, so I figured I’d share what I did.
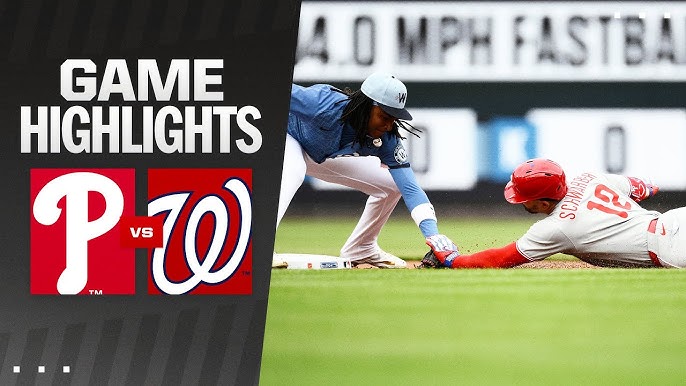
First off, I started by hitting up the usual sports data sites. You know, ESPN, *, that kind of thing. I was hoping to just scrape the box scores and game logs. But honestly, the way they format everything is a mess. Plus, they change their layouts all the time, which makes scraping a real headache.
I thought, “Alright, maybe there’s an API somewhere.” So I Googled around for “MLB API” and stumbled on a few options. Some of them were paid services, which I wasn’t really trying to do for a personal project. Then I found one that seemed promising – a free one with decent documentation.
The API needed some authentication, but luckily it wasn’t too complicated. I just had to sign up and get an API key. Once I had that, I started writing a quick Python script to pull the data. I used the requests
library because it’s super easy for making HTTP requests.
Here’s roughly what the code looked like (simplified, of course):
- Import the
requests
library. - Define the API endpoint for the Phillies vs. Nationals game – this took some digging to find the right URL format.
- Include my API key in the headers of the request.
- Make the GET request and check the status code. If it’s 200, that means it worked!
- Parse the JSON response – this is where things got a little hairy. The JSON was nested like crazy.
I kept getting errors about missing keys and stuff. Turns out, the API’s response format wasn’t exactly consistent. Sometimes certain fields would be missing depending on the game. I had to add a bunch of try...except
blocks to handle those cases gracefully. It was annoying, but gotta make the code robust, right?
Once I got the data parsing correctly, I started pulling out the stuff I cared about: runs, hits, RBIs, strikeouts – the usual stats. I dumped everything into a Pandas DataFrame. Pandas is a lifesaver for working with tabular data.
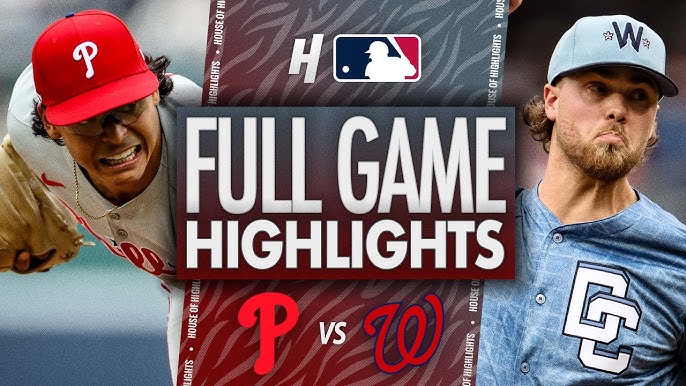
Next up, I wanted to do some simple analysis. Like, who had the most RBIs? What was the combined score? Just basic stuff. Pandas makes it easy to filter, group, and aggregate the data. A few lines of code, and I had some cool insights.
Finally, I visualized some of the data using Matplotlib. A simple bar chart of runs per inning, a scatter plot of batting average vs. RBIs. Nothing fancy, but it helped me see the story of the game at a glance.
Overall, it took me a few hours to get everything working. Dealing with messy APIs and inconsistent data formats is always a pain, but hey, that’s part of the fun, right? Plus, I learned a few new tricks along the way. Now I’ve got a nice little script I can reuse for future games.