Okay, so I wanted to mess around with creating a simple game, and I figured a good place to start would be getting some characters—actors—moving around on the screen. I’ve dabbled in game development before, but it’s been a while, so I’m basically starting from scratch here.
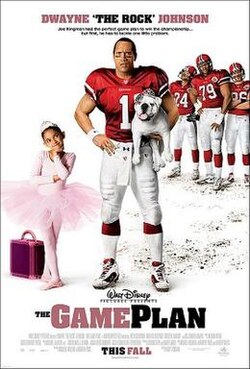
Getting Started
First, I needed a game engine. I didn’t want anything too complicated, just something to handle the basics. I went with a basic 2D game library in the language I wrote.
Once I had the engine set up, I created a new project. The initial setup was pretty straightforward – it gave me a basic window and a game loop that runs continuously.
Creating the Actor Class
Next, I needed a way to represent my actors. I decided to create a simple “Actor” class. This class would store things like the actor’s position, image, and any other relevant data.
Here’s what the basic class looked like:
- x, y: The position of the actor on the screen.
- image:The image used to represent the actor in the library I used.
- draw(): A method to draw the actor on the screen.
Implementing Movement
Now for the fun part – making the actors move! I added an “update” method to my Actor class. This method would be called every frame of the game loop, and it’s where I’d handle the actor’s movement logic.
I started simple: I just wanted the actor to move across the screen horizontally. Inside the “update” method, I incremented the actor’s x position by a small amount each frame.
Testing it Out
I created an instance of my Actor class, loaded a placeholder image, and added some code to the main game loop to call the actor’s “update” and “draw” methods each frame.
I ran the game, and… it worked! I had a little object moving across the screen. It was super basic, but it was a start.
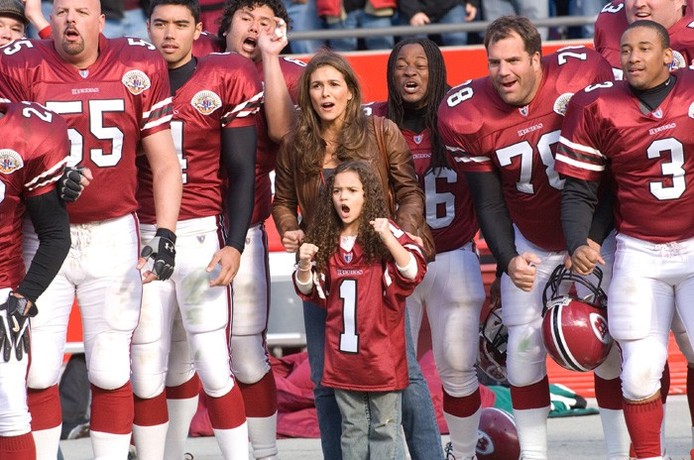
Adding Some Randomness
To make things a little more interesting, I decided to add some randomness to the actor’s movement. Instead of just moving horizontally, I wanted it to move in random directions.
So, instead of just incrementing the x position, I added a random number that can be generated by math function to both the x and y positions each frame.
I set bounds to ensure the picture not moving out of the window.
I ran the game again, and now my actor was bouncing around the screen randomly. It was way more interesting to watch!
Wrap Up
It’s still incredibly simple, but this little experiment taught me the basics of creating and moving actors in my chosen game engine. Next steps would be things like adding collision detection, user input, and maybe even some basic AI. But for now, I’m happy with my little bouncing actor!